When building out a web project in Symfony that needed CMS capabilities, we quickly turned to the excellent Sonata Admin Bundle. At it’s core, it provided us with a way to quickly generate an admin interface for all of the entities that need to be created and updated by site administrators. Sonata also provides a lot of handy extras, like media management, user management, and a suite of rich scaffolding tools to quickly integrate the bundles into any project.
One of the feature of the Sonata project that is especially useful is a new form type that allows easy selection of related entities, which looks something like this:
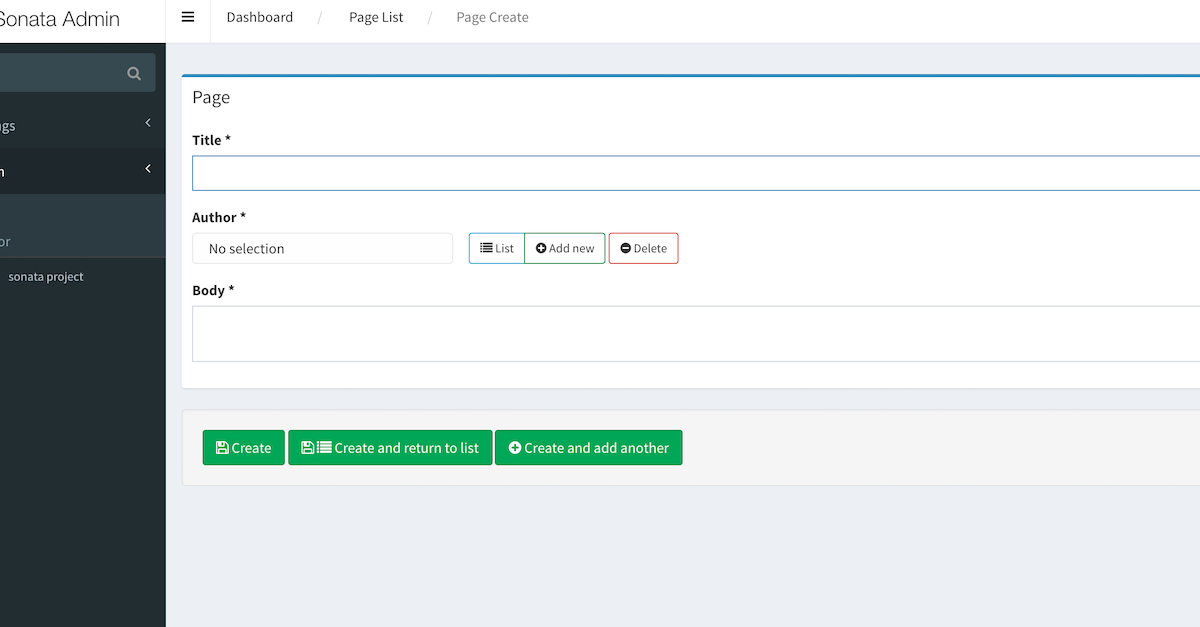
Clicking "list" will bring up the following model, which allows you to select from an existing list of Authors:
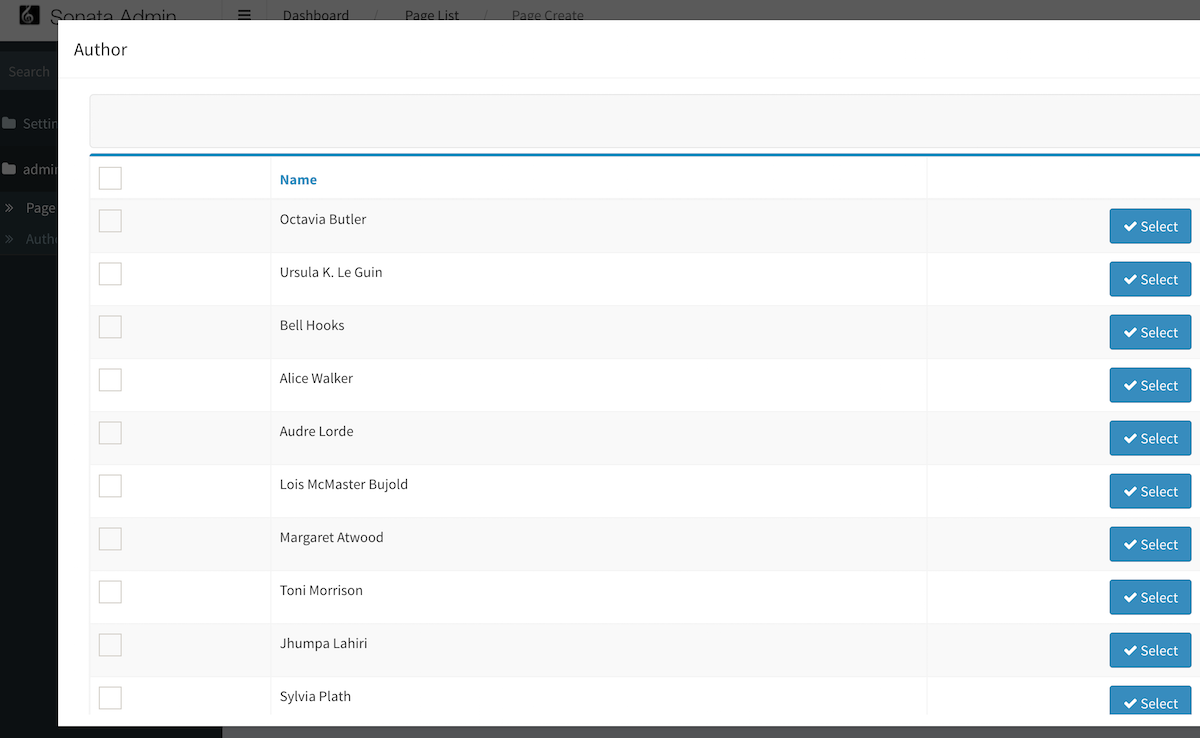
In this example, the Author entity is being selected in the Page entity edit form using the sonata_type_model_list
form type. Under the hood, this is simply loading the list view for the Author entity in a modal, which allows for easy searching and selecting of the related entity. Unfortunately, this also shows the batch operations for the list view, which are useless in this context.
The following is a solution that will remove those batch operations for this field type. It’s not ideal (I would have much rather used Admin Extensions, but unfortunately the required operations are not supported at the time of this writing).
Prerequisites
Altering Parent Entity Admin Class
The first step is to add a way to indicate to the Author (child) Admin class that we don’t want batch operations shown. We can do this form the form definition in the Page (parent) Admin class:
class PageAdmin extends BaseAdmin
{
...
/**
* @param FormMapper $formMapper
*/
protected function configureFormFields(FormMapper $formMapper)
{
$formMapper
->add('title')
->add('author', 'sonata_type_model_list', array(), array(
'link_parameters' => array(
'no_batch' => 1,
)))
->add('body')
;
}
…
}
The last argument to the author field, link_parameters
, will get added to the query string for the modal list view.
Altering Child Entity Admin Class
Next we need to respond in the Author (child) Admin class when the no_batch
parameter is passed in the request to remove batch/export operations (which cause the unneeded checkboxes to show). Fortunately, the AbstractAdmin
class allows batch and export operations to be defined by the implementing class, so we can respond with empty arrays when the no_batch
parameter is present:
class AuthorAdmin extends AbstractAdmin
...
protected function configureBatchActions($actions)
{
if ($this->hasRequest() && $this->getRequest()->get('no_batch')) {
return array();
}
else {
return parent::configureBatchActions($actions);
}
}
public function getExportFormats()
{
if ($this->hasRequest() && $this->getRequest()->get('no_batch')) {
return array();
}
else {
return parent::getExportFormats();
}
}
…
}
Since no batch or export operations are set for the admin interface when `no_batch` is present in the request, the checkboxes are no longer show:
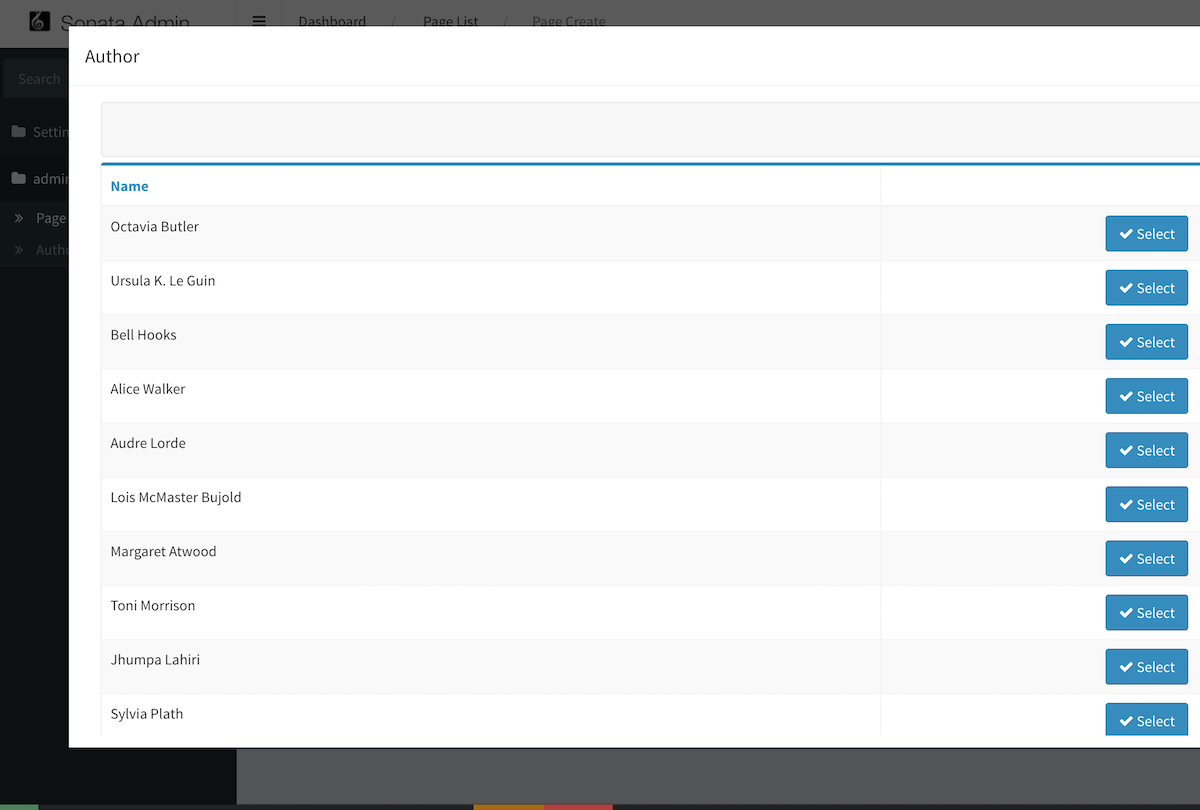
Note that this only prevents batch operations for the Author admin. The same steps will need to be applied to any other admin listings you want to integrate using the sonata_type_model_list
form type.