For this hackathon we created a product, YouToad, using Amplify (S3, React, Lambda) and AWS Elemental MediaConvert. Since we were using React and I wanted to learn JavaScript. We opted to use JavaScript for AWS Lambda as well (Check out our article "what is AWS Lambda?").
Objectives for the lambda:
- Get a trigger from S3.
- Parse the Event object.
- Trigger a Media Convert Job Create task using the SDK.
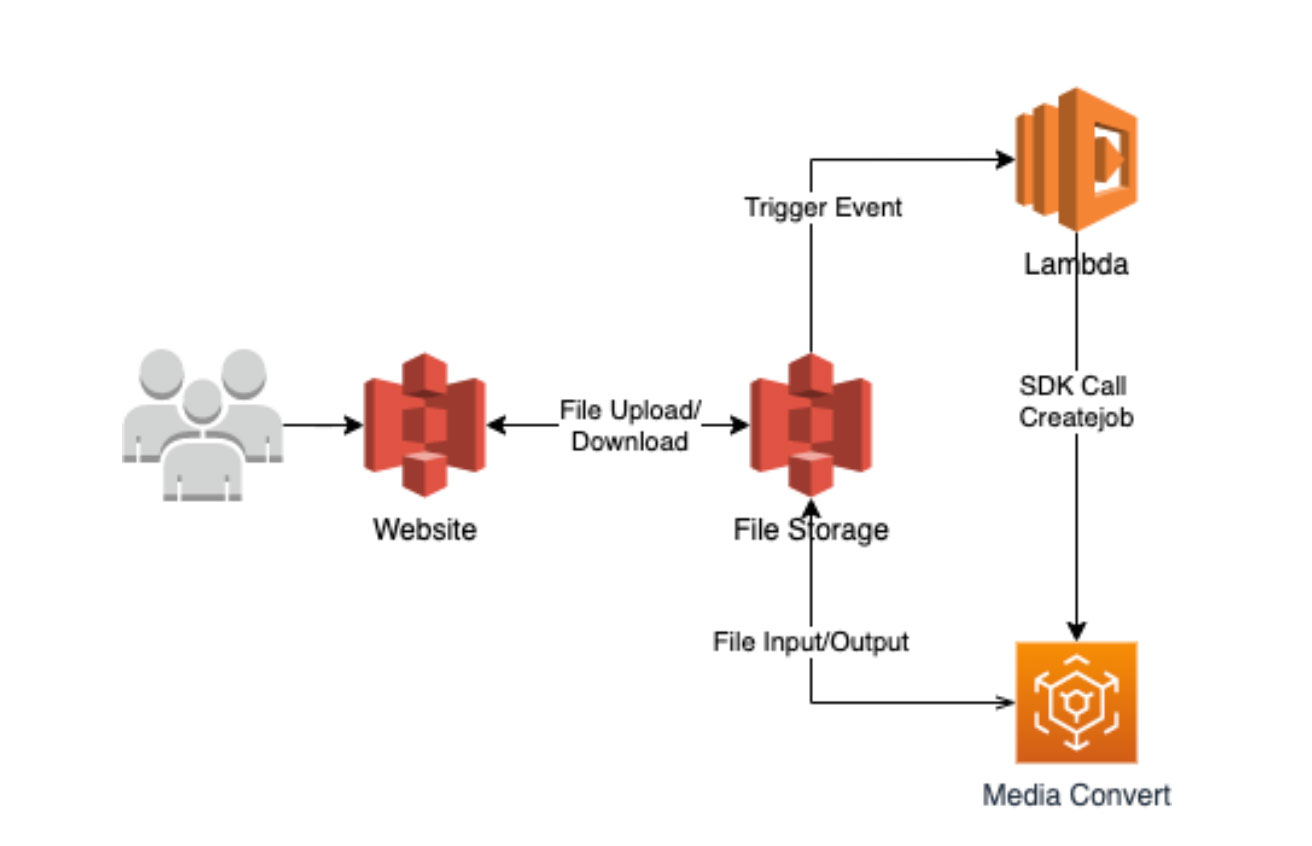
Before we dive into the code, a bit about my background in programming. Over the years, I’ve used PHP, Python, C++, C#, Pick, and probably a few other languages for different jobs. The right tool for the right job. Over the last few years I’ve learned more and more on Python because of how easy I find it to get started and how widespread the documentation is.
Let’s look at the code:
Here is the Javascript function. I’ve truncated the jobMetadata object because it’s large, the same in python and javascript, and copied out of a previous MediaConvert job.
We start with Imports. Required all modern languages, these are pretty standard. The two differences are that python gets all the SDK for AWS with a single import and Javascript uses several. This does allow javascript to be leaner since it normally runs in a web browser and has less resources to use.
JavaScript — Imports
// import entire SDK
var AWS = require('aws-sdk');
// import AWS object without services
var AWS = require('aws-sdk/global');
// import individual service
var S3 = require('aws-sdk/clients/s3');
Python — Imports
import json
import boto3
The Functions declaration is pretty similar and defined by AWS when creating a new function. (We may have added async to the javascript lambda.)
JavaScript — Function
exports.handler = async function(event, context) {
Python — Function
def lambda_handler(event, context):
The next two sections “Initialize Variables” and “Update Variables with Event data” are very similar. With the exception of syntax differences.
JavaScript — Initialize Variables
//mediaconvert template
var jobMetadata = {...};
// Set the Region
AWS.config.update({region: 'us-west-2'});
//get data out of s3 trigger
const bucket = event.Records[0].s3.bucket.name;
const key = event.Records[0].s3.object.key;
Python — Initialize Variables
#mediaconvert template
jobMetadata = {...}
#get data out of s3 trigger
bucket = event['Records'][0]['s3']['bucket']['name']
key = event['Records'][0]['s3']['object']['key']
#convert settings to json
json_settings = json.dumps(jobMetadata['Settings'])
JavaScript — Update Variables with Event Data
//mediaconvert template
jobMetadata.Settings.Inputs[0].FileInput = `s3://${bucket}/${key}`;
jobMetadata.Settings.OutputGroups[0].OutputGroupSettings.DashIsoGroupSettings.Destination = `s3://${bucket}/public/convert/`;
Python — Update Variables with Event Data
#update media convert template
jobMetadata['Settings']['Inputs'][0]['FileInput'] = 's3://' + bucket + '/' + key
jobMetadata['Settings']['OutputGroups'][0]['OutputGroupSettings']['DashIsoGroupSettings']['Destination'] = 's3://' + bucket + '/public/conver'
When we run Media Convert is when we really start to see the differences. Python handles the request in two lines that are relatively simple. Javascript works but requires setting different commands, building a request, and submitting it.
JavaScript — Media Convert
//MediaConvert
AWS.config.mediaconvert = {endpoint : 'https://mlboolfjb.mediaconvert.us-west-2.amazonaws.com'};
var mediaConvert = new AWS.MediaConvert({apiVersion: '2017-08-29'});
if (true) {
var request = mediaConvert.createJob(jobMetadata, function(data){
console.log(data);
},
function(error)
{
console.log(error)
}
);
var requestPromise = await request.promise();
console.log(requestPromise);
}
};
Python — Media Convert
#MediaConvert
client = boto3.client('mediaconvert', endpoint_url='https://mlboolfjb.mediaconvert.us-west-2.amazonaws.com')
response = client.create_job(
Role = jobMetadata['Role'],
Queue = jobMetadata['Queue'], Settings = json.loads(json_settings))
Closing is the closing curly bracket for Javascript and, though not required, a return statement for me to troubleshoot the responses.
JavaScript — Close
}
Python — Close
return {
'statusCode': 200,
'body': json.dumps(response)
}
In both cases the code is simple and easy to read. Most of the differences are syntax. Meaning: don’t for get your semi-colons.
The only significant difference was in calling Media Convert. I found the libraries were much better documented and more straightforward to use. I am biased and I am much more familiar with Python and the Boto library, so that probably played a role.
For simple tasks like this it doesn’t really matter what you chose. For me I’ll keep my default of python for now.